how to avoid triggering the OnClipboardChange event when the script itself modifies the clipboard? Topic is solved
Re: how to avoid triggering the OnClipboardChange event when the script itself modifies the clipboard?
The OnClipboardChange event will be triggered regardless, however, because you are defining your own callback function, you can test for a flag within the function. You simply need to set the flag immediately before filling the clipboard within your script. Run the following code and press F5 to see what happens when you set fill the clipboard programmatically, then copy some text with ^c to see the alternative.
Russ
Code: Select all
#Requires AutoHotkey v1.1.33+
ClipFlag := False
OnClipBoardChange("MyClipFunc")
Return
F5::
ClipFlag := True
ClipBoard := "Clipboard set by program"
Return
F10:: ExitApp
MyClipFunc(DataType){
Global ClipFlag
If (ClipFlag) {
ClipFlag := False
MsgBox % Clipboard
} Else {
MsgBox % "Clipboard set outside of program"
}
}
Re: how to avoid triggering the OnClipboardChange event when the script itself modifies the clipboard?
You can actually check who set the clipboard last, and filter based on that. E.g.
Code: Select all
#Requires AutoHotkey v1.1.33+
OnClipboardChange("MyClipFunc")
return
F5::Clipboard := "Clipboard set by program"
MyClipFunc(DataType) {
if (DllCall("GetClipboardOwner") == A_ScriptHwnd)
return
MsgBox Clipboard set outside of program
}
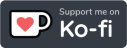
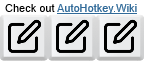



Re: how to avoid triggering the OnClipboardChange event when the script itself modifies the clipboard?
@geek - nice! Same principle, but saves having to remember to set the flag every time your are going to fill the clipboard.
I looked up the function in Win32 for fun. I am always amazed at how people can find anything in that API. If you don't happen to know the exact function name to search for, you would need to drill down into the API. GetClipboardOwner() is found at Win32/API/Data Exchange/Winuser.h. Everything makes sense up to the Winuser.h part. It seems to be nothing but clipboard functions. Who would think that a section called Winuser would be nothing but clipboard functions? I sure wouldn't. Anyway, thanks for the assist!
Russ
I looked up the function in Win32 for fun. I am always amazed at how people can find anything in that API. If you don't happen to know the exact function name to search for, you would need to drill down into the API. GetClipboardOwner() is found at Win32/API/Data Exchange/Winuser.h. Everything makes sense up to the Winuser.h part. It seems to be nothing but clipboard functions. Who would think that a section called Winuser would be nothing but clipboard functions? I sure wouldn't. Anyway, thanks for the assist!
Russ
Re: how to avoid triggering the OnClipboardChange event when the script itself modifies the clipboard?
"Disable" OnClipboardChange (stop callback)
Technique #3:
OnClipboardChange():
OnClipboardChange(Callback [, AddRemove])
If needed, the AddRemove parameter can be used to avoid calling the callback.
Technique #3:
OnClipboardChange():
OnClipboardChange(Callback [, AddRemove])
If needed, the AddRemove parameter can be used to avoid calling the callback.
If omitted, it defaults to 1. Otherwise, specify one of the following numbers:
1 = Call the callback after any previously registered callbacks.
-1 = Call the callback before any previously registered callbacks.
0 = Do not call the callback.
Code: Select all
; This script shows how to "disable" OnClipboardChange (stop callback)
#Requires AutoHotkey v1.1.33
#Persistent
OnClipboardChange("done")
done(dataType) {
OnClipboardChange("done", False)
Clipboard := "Clipboard:`n`n" Clipboard
MsgBox 64, Information, % Clipboard
OnClipboardChange("done")
}
Re: how to avoid triggering the OnClipboardChange event when the script itself modifies the clipboard? Topic is solved
thank you sirs all three methods are effective