Hello,
Lets say we have a simple GUI.
The question is:
How can we RESIZE it with ALL its contents resized (zoomed in or zoomed out) accordingly?
The Gui, +resize is not resize ALL GUI contents - it just add a resize of the GUI Window.
I see the relevant topic at:
viewtopic.php?t=95178
My question is:
Is there an EASY (not complicated) method to do it?
For example something like:
Gui, +resizeALL ???
Resize GUI with ALL its contents
Re: Resize GUI with ALL its contents
There is no "easy solution" because "resize" is a blanket term.
Some examples.
.
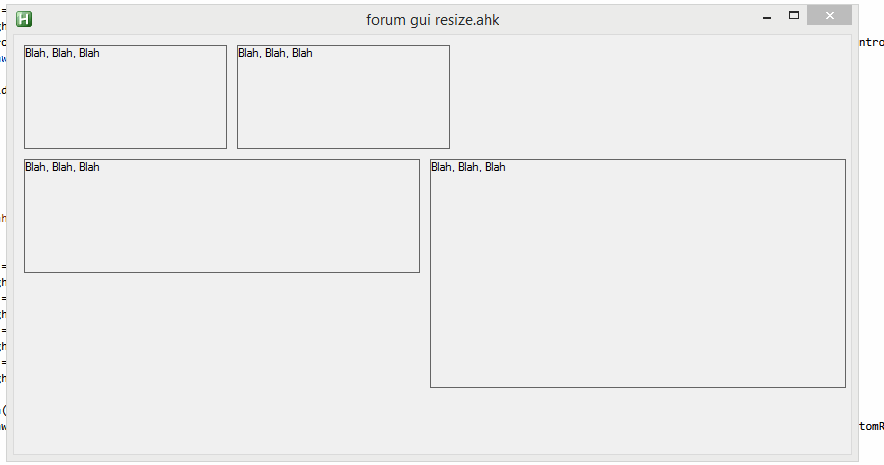
.
viewtopic.php?t=95178#p423175
.
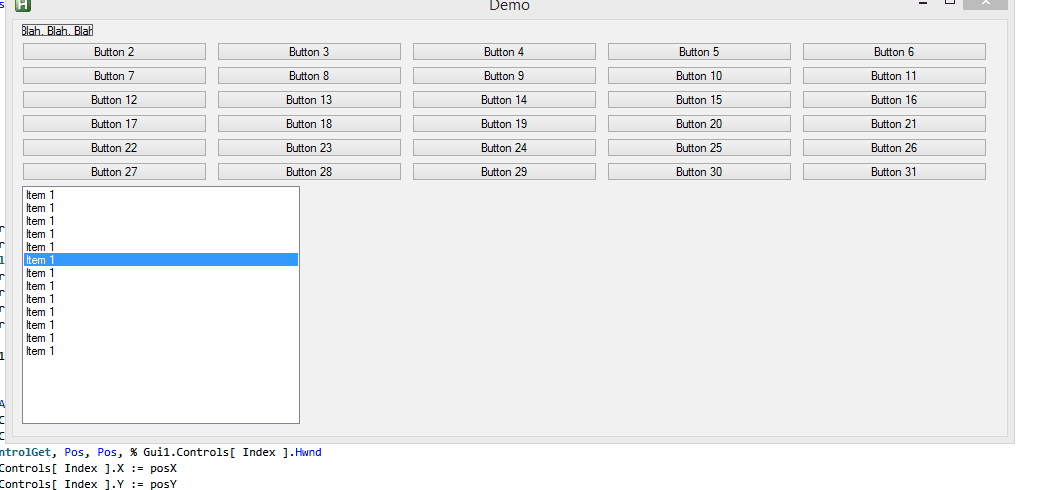
.
viewtopic.php?t=95178#p423178
.

.
viewtopic.php?f=76&t=119635&p=531550#p530844
.

.
viewtopic.php?f=76&t=119635&p=531550#p530862
. .
The best way to do that is to create an object for each control { X: "" , Y: "" , W: "" , H: "" } and apply your multiplier to each (Font size is a thing
).
. .
Some examples.
.
.
viewtopic.php?t=95178#p423175
.
.
viewtopic.php?t=95178#p423178
.
.
viewtopic.php?f=76&t=119635&p=531550#p530844
.
.
viewtopic.php?f=76&t=119635&p=531550#p530862
. .
This suggests that you are talking about scaling the gui which is largely just applying a multiplier to every position and size.How can we RESIZE it with ALL its contents resized (zoomed in or zoomed out) accordingly?
The best way to do that is to create an object for each control { X: "" , Y: "" , W: "" , H: "" } and apply your multiplier to each (Font size is a thing

. .
Re: Resize GUI with ALL its contents
Thank you very much!
Re: Resize GUI with ALL its contents
Here is a Gui class that has scaling.
. .
This works with the normal set of gui controls.
If you use controls such as ListViews, etc. you will have to do scaling for those controls separately.
( OriginalSize -> OriginalSize * Scale )
Here is the class. Just paste it at the bottom of any script you want to use it in.
***Make sure that you turn off the default scaling ( "-DPIScale" )
GUI CLASS
Here is an example of creating a new window.
***Make sure that you turn off the default scaling ( "-DPIScale" )
Gui Syntax Example 1
Here is a second example.
***Make sure that you turn off the default scaling ( "-DPIScale" )
Gui Syntax Example 2
Here is an example of using the mouse wheel hotkey to change the scale. (uses the two example windows from above)
Scaling Example
Lastly. Here is my full test script.
Full Working Example
. .
This works with the normal set of gui controls.
If you use controls such as ListViews, etc. you will have to do scaling for those controls separately.
( OriginalSize -> OriginalSize * Scale )
Here is the class. Just paste it at the bottom of any script you want to use it in.
***Make sure that you turn off the default scaling ( "-DPIScale" )
GUI CLASS
Code: Select all
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
class Gui {
static Index := 0 , Windows := [] , Handles := []
;Written By: Hellbent
;Date: Sept 9th, 2021
;Last Edit: Oct 16th 2023
;Class to create Easy Scale windows
__New( Options := "+AlwaysOnTop" , Title := "" ){
local hwnd
;********************
Gui, New, % Options " +Hwndhwnd" , % Title
This.Hwnd := hwnd
;********************
This.Controls := {}
This.ControlArray := []
This.Handles := []
;********************
This.FontType := "Arial"
This.FontSize := 10
This.FontColor := "Black"
This.FontOptions := ""
This.Font()
;********************
This.Color := "F0F0F0"
This.ControlColor := "FFFFFF"
This.SetColors()
;********************
Gui.Handles[ This.Hwnd ] := Gui.Windows[ ++Gui.Index ] := This
This.Show( "Hide w1 h1 " )
}
Show( Options := "" , Title := "" ){
Gui, % This.Hwnd ":Show" , % Options , % Title
}
Hide( Name := 1 ){
Gui, % Name ":Hide"
}
Enable(){
Gui, % This.Hwnd ":-Disabled"
}
Disable(){
Gui, % This.Hwnd ":+Disabled"
}
Destroy( Name := 1 ){
Try
Gui, % This.Hwnd ":Destroy"
}
__Delete(){
Try
Gui, % This.Hwnd ":Destroy"
}
Get( Control , SubCommand := "" ){
local output
GuiControlGet, output, % This.Hwnd ":" SubCommand , % Control
return output
}
Set( Control , Value := "" , SubCommand := "" ){
GuiControl, % This.Hwnd ":" SubCommand , % Control , % Value
}
Margin( x := 10 , y := 10 ){
Gui, % This.Hwnd ":Margin", % x , % y
}
SetColors( Color1 := "" , Color2 := "" ){
if( Color1 != "" )
This.Color := Color1
if( Color2 != "" )
This.ControlColor := Color2
Gui, % This.Hwnd ":Color", % This.Color , % This.ControlColor
}
WindowPosition(){
local x , y , w , h
WinGetPos, x , y , w , h , % "ahk_id " This.Hwnd
return { X: x , Y: y , W: w , H: h }
}
ControlPosition( Hwnd ){
local pos , posX , posY , PosW , PosH
GuiControlGet, pos , % This.Hwnd ":pos", % Hwnd
if( This.Handles[ hwnd ].Index ){
This.Handles[ hwnd ].Position := { X: posX , Y: posY , W: posW , H: posH }
return
}else{
return { X: posX , Y: posY , W: posW , H: posH }
}
}
Add( Type := "Button" , Options := "" , Display := "" , ControlName := "" , ScaleMode := "" , rows := "" ){ ;scaleMode 1: scale via font size. 2= scale via controls size
static ScaleModeList := { "Text": 1 , "Edit": 1 , "Button": 1 , "DDL": 2 , "DropDownList": 2 , "ListBox": 1 , "CheckBox": 1 }
local hwnd
Gui, % This.Hwnd ":Add", % Type, % Options " hwndhwnd " , % Display
if( ControlName != "" ){
This.Controls[ ControlName ] := {}
This.Controls[ ControlName ].Name := ControlName
This.Controls[ ControlName ].Hwnd := hwnd
This.Controls[ ControlName ].Type := type
This.Handles[ hwnd ] := This.Controls[ ControlName ]
This.ControlArray.Push( This.Controls[ ControlName ] )
}else{
This.Controls[ hwnd ] := {}
This.Controls[ hwnd ].Name := hwnd
This.Controls[ hwnd ].Type := Type
This.Controls[ hwnd ].Hwnd := hwnd
This.Handles[ hwnd ] := This.Controls[ hwnd ]
This.ControlArray.Push( This.Controls[ hwnd ] )
}
if( ScaleMode != "" )
This.Handles[ hwnd ].ScaleMode := ScaleMode
else
This.Handles[ hwnd ].ScaleMode := ScaleModeList[ type ]
if( This.Handles[ hwnd ].ScaleMode = "" )
This.Handles[ hwnd ].ScaleMode := 1
This.Handles[ hwnd ].Index := This.ControlArray.Length()
This.ControlPosition( Hwnd )
This.Handles[ hwnd ].Font := { Type: This.FontType , Size: This.FontSize , Color: This.FontColor , Options: This.FontOptions }
if( rows != "" )
This.Handles[ hwnd ].Rows := rows
}
SetFont( Size := "" , color := "" , Options := "" , Type := "" ){
if( Size != "" )
This.FontSize := Size
if( color != "" )
This.FontColor := Color
if( Options != "" )
This.FontOptions := Options
if( Type != "" )
This.FontType := Type
Gui, % This.Hwnd ":Font", % "norm s" ( This.FontSize * A_ScreenDPI / 96 ) " c" This.FontColor " " This.FontOptions , % This.FontType
}
Options( Options ){
Gui, % This.Hwnd ":" Options
}
Bind( Control , Method , BoundObject := "" ){
local fn
if( BoundObject = "Label" )
fn := Method
else if( BoundObject != "" )
fn := Func( Method ).Bind( This , Control , BoundObject )
else
fn := Func( Method ).Bind( This , Control )
GuiControl, % This.Hwnd ":+g", % Control, % fn
}
ScaleControl( ControlHwnd , scalefactor , mode := 1 ){
if( mode = 1 ){
cc := This.Handles[ ControlHwnd ].Position
x := cc.X * scaleFactor
y := cc.Y * scaleFactor
w := cc.W * scaleFactor
h := cc.H * scaleFactor
This.SetControlFont( controlhwnd , , , , , scalefactor )
This.Set( ControlHwnd , "X" x " y" y " w " w " h" h , "MoveDraw" )
}else if( mode = 2 ){
cc := This.Handles[ ControlHwnd ].Position
x := cc.X * scaleFactor
y := cc.Y * scaleFactor
w := cc.W * scaleFactor
h := ( 16 * This.Handles[ ControlHwnd ].Rows ) * scaleFactor
This.SetControlFont( controlhwnd , , , , , scalefactor )
This.Set( ControlHwnd , "X" x " y" y " w " w " h" h , "MoveDraw" )
}
}
SetControlFont( controlhwnd , Size := "" , color := "" , Options := "" , Type := "" , Scale := 1 ){
cc := This.Handles[ controlhwnd ].Font
if( Size != "" ){
cc.Size := Size
Size /= A_ScreenDPI / 96
Size *= Scale
}else{
Size := ( cc.Size / ( A_ScreenDPI / 96 ) ) * Scale
}
if( color != "" )
cc.Color := Color
else
Color := cc.Color
if( Options != "" )
cc.Options := Options
else
Options := cc.Options
if( Type != "" )
cc.Type := Type
else
Type := cc.Type
Gui, % This.Hwnd ":Font" , % " norm s" size " c" color " " options , % Type
This.Set( controlhwnd ,, "Font" )
}
ScaleAllControls( scale := 1 ){
for k, v in This.ControlArray {
cc := This.ControlArray[ k ]
This.ScaleControl( cc.Hwnd , Scale , mode := cc.ScaleMode )
}
}
}
***Make sure that you turn off the default scaling ( "-DPIScale" )
Gui Syntax Example 1
Code: Select all
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
Gui1 := New Gui( "+AlwaysOnTop -DpiScale " , "Gui Scale" )
Gui1.Margin( 10 , 10 )
Gui1.SetFont( 8 , "White" , "Bold" , "Microsoft YaHei" )
Gui1.SetColors( "22262a" )
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;********************************************************************************************************
;Method Name: Add
;Purpose: Add a new control to a window
;First Parameter: The name of the window object ( "Gui1" )
;Second Parameter: The name of the method to call ( "Add" )
;Third Parameter: The type of control to add ( eg. "Button" , "Text" , "Edit" ) [ < Control Type > ]
;Forth Parameter: The options to use when creating the new control ( eg. "xm ym w200 h200 backgroundTrans gLable " ) [ < Control Options > ]
;Fifth Parameter: The display value for the new control ( includes lists ) [ < Display Value > ]
;Sixth Parameter: ( Optional ) The name to give the controls object reference ( ex. "SaveButton" "LastNameEdit" ) [ < Control Name > ] *Optional
;Seventh Parameter: ( Optional ) The mode to use when scaling ( [ h * scale ] vs. [ fontsize * scale + Dummycontrol ] ) [ < Scale Mode > ] *Optional
;********************************************************************************************************
; --->>> Gui1.Add( < Control Type > , < Control Options > , < Display Value > , < Control Name > , < Scale Mode > , < Rows > )
;__________________________________________________________________________
Gui1.Add( "Picture" , "xm w300 h-1" , "C:\Users\CivRe\OneDrive\Desktop\AHK Tools\Color Picker Mini\Screen Shots\20231017121645.png" )
Gui1.Add( "Button" , "xm w120 r1" , "Show" )
Gui1.Add( "Button" , "xm w120 r1" , "Hide" )
Gui1.Add( "Button" , "x+m w120 r1" , "Delete" )
Gui1.Add( "Checkbox" , "xm w120 r1" , "Turn On / Off" )
Gui1.Add( "Picture" , "xm w300 h-1" , "C:\Users\CivRe\OneDrive\Desktop\AHK Tools\Color Picker Mini\Screen Shots\20231017121722.png" )
;********************************************************************************************************
; --->>> Gui1.Font( < Font Size > , < Font Color > , < Font Options > , < Font Type > )
;__________________________________________________________________________
;~ Gui1.Font( 18 , "Black" , "Bold" , "Microsoft YaHei" )
;********************************************************************************************************
; --->>> Gui1.Add( < Control Type > , < Control Options > , < Display Value > , < Control Name > , < Scale Mode > , < Rows > )
;__________________________________________________________________________
Gui1.Add( "DDL" , "xm w120 r5" , "Show||Hide|I3|I4|I5" , , 2 , 7 )
Gui1.Add( "Edit" , "xm w120 r1" , "Show" )
Gui1.Add( "ListBox" , "xm w120 r5" , "Show|Hide" )
;********************************************************************************************************
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
Gui1.Scale := 1.5
Gui1.ScaleAllControls( Gui1.Scale )
Gui1.Margin( 10 * Gui1.Scale , 10 * Gui1.Scale )
Gui1.Show( "AutoSize x200 y100" )
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
***Make sure that you turn off the default scaling ( "-DPIScale" )
Gui Syntax Example 2
Code: Select all
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
Gui2 := New Gui( "+AlwaysOnTop -DpiScale " , "Gui Scale 2" )
Gui2.Margin( 10 , 10 )
Gui2.SetFont( 8 , "White" , "Bold" , "Microsoft YaHei" )
Gui2.SetColors( "22262a" )
y := 10 , w := 120
Index := 0
Loop, 6 {
x := 10
Loop, 6 {
Gui2.Add( "Button" , "x" x " y" y " w" 120 " r1 " , "Button: " ++Index , "Button_" Index )
x += w + 10
}
y += Gui2.Controls[ "Button_" Index ].Position.H + 10
}
Gui2.Scale := .7
Gui2.ScaleAllControls( Gui2.Scale )
Gui2.Margin( 10 * Gui2.Scale , 10 * Gui2.Scale )
Gui2.Show( "AutoSize" )
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
Here is an example of using the mouse wheel hotkey to change the scale. (uses the two example windows from above)
Scaling Example
Code: Select all
~$WheelUp:: ;{
name := ""
MouseGetPos,,, win
if( win = Gui1.Hwnd )
name := "Gui1"
else if( win = Gui2.Hwnd )
name := "Gui2"
if( Name ){
(%Name%).Scale += 0.1
(%Name%).ScaleAllControls( (%Name%).Scale )
(%Name%).Margin( 10 * (%Name%).Scale , 10 * (%Name%).Scale )
(%Name%).Show( "AutoSize" )
}
ToolTip, % "Scale:`n" (%Name%).Scale
SetTimer, TipsOff, -1000
return
;}
Full Working Example
Code: Select all
#SingleInstance Force
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
Gui1 := New Gui( "+AlwaysOnTop -DpiScale " , "Gui Scale" )
Gui1.Margin( 10 , 10 )
Gui1.SetFont( 8 , "White" , "Bold" , "Microsoft YaHei" )
Gui1.SetColors( "22262a" )
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;********************************************************************************************************
;Method Name: Add
;Purpose: Add a new control to a window
;First Parameter: The name of the window object ( "Gui1" )
;Second Parameter: The name of the method to call ( "Add" )
;Third Parameter: The type of control to add ( eg. "Button" , "Text" , "Edit" ) [ < Control Type > ]
;Forth Parameter: The options to use when creating the new control ( eg. "xm ym w200 h200 backgroundTrans gLable " ) [ < Control Options > ]
;Fifth Parameter: The display value for the new control ( includes lists ) [ < Display Value > ]
;Sixth Parameter: ( Optional ) The name to give the controls object reference ( ex. "SaveButton" "LastNameEdit" ) [ < Control Name > ] *Optional
;Seventh Parameter: ( Optional ) The mode to use when scaling ( [ h * scale ] vs. [ fontsize * scale + Dummycontrol ] ) [ < Scale Mode > ] *Optional
;********************************************************************************************************
; --->>> Gui1.Add( < Control Type > , < Control Options > , < Display Value > , < Control Name > , < Scale Mode > , < Rows > )
;__________________________________________________________________________
Gui1.Add( "Picture" , "xm w300 h-1" , "C:\Users\CivRe\OneDrive\Desktop\AHK Tools\Color Picker Mini\Screen Shots\20231017121645.png" )
Gui1.Add( "Button" , "xm w120 r1" , "Show" )
Gui1.Add( "Button" , "xm w120 r1" , "Hide" )
Gui1.Add( "Button" , "x+m w120 r1" , "Delete" )
Gui1.Add( "Checkbox" , "xm w120 r1" , "Turn On / Off" )
Gui1.Add( "Picture" , "xm w300 h-1" , "C:\Users\CivRe\OneDrive\Desktop\AHK Tools\Color Picker Mini\Screen Shots\20231017121722.png" )
;********************************************************************************************************
; --->>> Gui1.Font( < Font Size > , < Font Color > , < Font Options > , < Font Type > )
;__________________________________________________________________________
;~ Gui1.Font( 18 , "Black" , "Bold" , "Microsoft YaHei" )
;********************************************************************************************************
; --->>> Gui1.Add( < Control Type > , < Control Options > , < Display Value > , < Control Name > , < Scale Mode > , < Rows > )
;__________________________________________________________________________
Gui1.Add( "DDL" , "xm w120 r5" , "Show||Hide|I3|I4|I5" , , 2 , 7 )
Gui1.Add( "Edit" , "xm w120 r1" , "Show" )
Gui1.Add( "ListBox" , "xm w120 r5" , "Show|Hide" )
;********************************************************************************************************
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
Gui1.Scale := 1.5
Gui1.ScaleAllControls( Gui1.Scale )
Gui1.Margin( 10 * Gui1.Scale , 10 * Gui1.Scale )
Gui1.Show( "AutoSize x200 y100" )
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
Gui2 := New Gui( "+AlwaysOnTop -DpiScale " , "Gui Scale 2" )
Gui2.Margin( 10 , 10 )
Gui2.SetFont( 8 , "White" , "Bold" , "Microsoft YaHei" )
Gui2.SetColors( "22262a" )
y := 10 , w := 120
Index := 0
Loop, 6 {
x := 10
Loop, 6 {
Gui2.Add( "Button" , "x" x " y" y " w" 120 " r1 " , "Button: " ++Index , "Button_" Index )
x += w + 10
}
y += Gui2.Controls[ "Button_" Index ].Position.H + 10
}
Gui2.Scale := .7
Gui2.ScaleAllControls( Gui2.Scale )
Gui2.Margin( 10 * Gui2.Scale , 10 * Gui2.Scale )
Gui2.Show( "AutoSize" )
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
return
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
GuiClose: ;{
GuiContextMenu:
*ESC::ExitApp
;}
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
~$WheelUp:: ;{
name := ""
MouseGetPos,,, win
if( win = Gui1.Hwnd )
name := "Gui1"
else if( win = Gui2.Hwnd )
name := "Gui2"
if( Name ){
(%Name%).Scale += 0.1
(%Name%).ScaleAllControls( (%Name%).Scale )
(%Name%).Margin( 10 * (%Name%).Scale , 10 * (%Name%).Scale )
(%Name%).Show( "AutoSize" )
}
ToolTip, % "Scale:`n" (%Name%).Scale
SetTimer, TipsOff, -1000
return
;}
~$WheelDown:: ;{
name := ""
MouseGetPos,,, win
if( win = Gui1.Hwnd )
name := "Gui1"
else if( win = Gui2.Hwnd )
name := "Gui2"
if( Name ){
( ( (%Name%).Scale -= 0.1 ) < 0.3 ) ? ( (%Name%).Scale := 0.3 )
(%Name%).ScaleAllControls( (%Name%).Scale )
(%Name%).Margin( 10 * (%Name%).Scale , 10 * (%Name%).Scale )
(%Name%).Show( "AutoSize" )
}
ToolTip, % "Scale:`n" (%Name%).Scale
SetTimer, TipsOff, -1000
return
;}
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
F1:: ;{
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;~ list := [ 0.4 , 0.5 , 0.6 , 0.7 , 0.8 , 0.9 , 1 , 1.1 , 1.2 , 1.25 , 1.3 , 1.4 , 1.5 , 1.6 , 1.7 , 1.8 , 1.9 , 2 , 2.5 , 3 , 5 ]
list := [ 1 , 1.01 , 1.02 , 1.03 , 1.04 , 1.05 , 1.06 , 1.07 , 1.08 , 1.09 , 1.1 ]
;~ scale := List[ Random( 1 , list.Length() ) ]
scale := Random( 0 , 1 ) "." Random( 4 , 9 ) Random( 0 , 9 )
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
for k, v in Gui2.Handles {
cc := Gui2.Handles[ k ]
Gui2.ScaleControl( cc.Hwnd , scale , mode := cc.ScaleMode )
}
Gui2.Margin( 10 * Scale , 10 * Scale )
Gui2.Show( "AutoSize" )
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
for k, v in Gui1.Handles {
cc := Gui1.Handles[ k ]
Gui1.ScaleControl( cc.Hwnd , scale , mode := cc.ScaleMode )
}
Gui1.Margin( 10 * Scale , 10 * Scale )
Gui1.Show( "AutoSize" )
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
ToolTip, % "Scale:`n" scale
SetTimer, TipsOff, -1000
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
return
;}
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
Random( Min := 0 , Max := 100 ){
local out
Random, out , Min , Max
return out
}
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
TipsOff: ;{
ToolTip
return
;}
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
;|<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>||<<<---(0)_(0)--->>>|
class Gui {
static Index := 0 , Windows := [] , Handles := []
;Written By: Hellbent
;Date: Sept 9th, 2021
;Last Edit: Oct 16th 2023
;Class to create Easy Scale windows
__New( Options := "+AlwaysOnTop" , Title := "" ){
local hwnd
;********************
Gui, New, % Options " +Hwndhwnd" , % Title
This.Hwnd := hwnd
;********************
This.Controls := {}
This.ControlArray := []
This.Handles := []
;********************
This.FontType := "Arial"
This.FontSize := 10
This.FontColor := "Black"
This.FontOptions := ""
This.Font()
;********************
This.Color := "F0F0F0"
This.ControlColor := "FFFFFF"
This.SetColors()
;********************
Gui.Handles[ This.Hwnd ] := Gui.Windows[ ++Gui.Index ] := This
This.Show( "Hide w1 h1 " )
}
Show( Options := "" , Title := "" ){
Gui, % This.Hwnd ":Show" , % Options , % Title
}
Hide( Name := 1 ){
Gui, % Name ":Hide"
}
Enable(){
Gui, % This.Hwnd ":-Disabled"
}
Disable(){
Gui, % This.Hwnd ":+Disabled"
}
Destroy( Name := 1 ){
Try
Gui, % This.Hwnd ":Destroy"
}
__Delete(){
Try
Gui, % This.Hwnd ":Destroy"
}
Get( Control , SubCommand := "" ){
local output
GuiControlGet, output, % This.Hwnd ":" SubCommand , % Control
return output
}
Set( Control , Value := "" , SubCommand := "" ){
GuiControl, % This.Hwnd ":" SubCommand , % Control , % Value
}
Margin( x := 10 , y := 10 ){
Gui, % This.Hwnd ":Margin", % x , % y
}
SetColors( Color1 := "" , Color2 := "" ){
if( Color1 != "" )
This.Color := Color1
if( Color2 != "" )
This.ControlColor := Color2
Gui, % This.Hwnd ":Color", % This.Color , % This.ControlColor
}
WindowPosition(){
local x , y , w , h
WinGetPos, x , y , w , h , % "ahk_id " This.Hwnd
return { X: x , Y: y , W: w , H: h }
}
ControlPosition( Hwnd ){
local pos , posX , posY , PosW , PosH
GuiControlGet, pos , % This.Hwnd ":pos", % Hwnd
if( This.Handles[ hwnd ].Index ){
This.Handles[ hwnd ].Position := { X: posX , Y: posY , W: posW , H: posH }
return
}else{
return { X: posX , Y: posY , W: posW , H: posH }
}
}
Add( Type := "Button" , Options := "" , Display := "" , ControlName := "" , ScaleMode := "" , rows := "" ){ ;scaleMode 1: scale via font size. 2= scale via controls size
static ScaleModeList := { "Text": 1 , "Edit": 1 , "Button": 1 , "DDL": 2 , "DropDownList": 2 , "ListBox": 1 , "CheckBox": 1 }
local hwnd
Gui, % This.Hwnd ":Add", % Type, % Options " hwndhwnd " , % Display
if( ControlName != "" ){
This.Controls[ ControlName ] := {}
This.Controls[ ControlName ].Name := ControlName
This.Controls[ ControlName ].Hwnd := hwnd
This.Controls[ ControlName ].Type := type
This.Handles[ hwnd ] := This.Controls[ ControlName ]
This.ControlArray.Push( This.Controls[ ControlName ] )
}else{
This.Controls[ hwnd ] := {}
This.Controls[ hwnd ].Name := hwnd
This.Controls[ hwnd ].Type := Type
This.Controls[ hwnd ].Hwnd := hwnd
This.Handles[ hwnd ] := This.Controls[ hwnd ]
This.ControlArray.Push( This.Controls[ hwnd ] )
}
if( ScaleMode != "" )
This.Handles[ hwnd ].ScaleMode := ScaleMode
else
This.Handles[ hwnd ].ScaleMode := ScaleModeList[ type ]
if( This.Handles[ hwnd ].ScaleMode = "" )
This.Handles[ hwnd ].ScaleMode := 1
This.Handles[ hwnd ].Index := This.ControlArray.Length()
This.ControlPosition( Hwnd )
This.Handles[ hwnd ].Font := { Type: This.FontType , Size: This.FontSize , Color: This.FontColor , Options: This.FontOptions }
if( rows != "" )
This.Handles[ hwnd ].Rows := rows
}
SetFont( Size := "" , color := "" , Options := "" , Type := "" ){
if( Size != "" )
This.FontSize := Size
if( color != "" )
This.FontColor := Color
if( Options != "" )
This.FontOptions := Options
if( Type != "" )
This.FontType := Type
Gui, % This.Hwnd ":Font", % "norm s" ( This.FontSize * A_ScreenDPI / 96 ) " c" This.FontColor " " This.FontOptions , % This.FontType
}
Options( Options ){
Gui, % This.Hwnd ":" Options
}
Bind( Control , Method , BoundObject := "" ){
local fn
if( BoundObject = "Label" )
fn := Method
else if( BoundObject != "" )
fn := Func( Method ).Bind( This , Control , BoundObject )
else
fn := Func( Method ).Bind( This , Control )
GuiControl, % This.Hwnd ":+g", % Control, % fn
}
ScaleControl( ControlHwnd , scalefactor , mode := 1 ){
if( mode = 1 ){
cc := This.Handles[ ControlHwnd ].Position
x := cc.X * scaleFactor
y := cc.Y * scaleFactor
w := cc.W * scaleFactor
h := cc.H * scaleFactor
This.SetControlFont( controlhwnd , , , , , scalefactor )
This.Set( ControlHwnd , "X" x " y" y " w " w " h" h , "MoveDraw" )
}else if( mode = 2 ){
cc := This.Handles[ ControlHwnd ].Position
x := cc.X * scaleFactor
y := cc.Y * scaleFactor
w := cc.W * scaleFactor
h := ( 16 * This.Handles[ ControlHwnd ].Rows ) * scaleFactor
This.SetControlFont( controlhwnd , , , , , scalefactor )
This.Set( ControlHwnd , "X" x " y" y " w " w " h" h , "MoveDraw" )
}
}
SetControlFont( controlhwnd , Size := "" , color := "" , Options := "" , Type := "" , Scale := 1 ){
cc := This.Handles[ controlhwnd ].Font
if( Size != "" ){
cc.Size := Size
Size /= A_ScreenDPI / 96
Size *= Scale
}else{
Size := ( cc.Size / ( A_ScreenDPI / 96 ) ) * Scale
}
if( color != "" )
cc.Color := Color
else
Color := cc.Color
if( Options != "" )
cc.Options := Options
else
Options := cc.Options
if( Type != "" )
cc.Type := Type
else
Type := cc.Type
Gui, % This.Hwnd ":Font" , % " norm s" size " c" color " " options , % Type
This.Set( controlhwnd ,, "Font" )
}
ScaleAllControls( scale := 1 ){
for k, v in This.ControlArray {
cc := This.ControlArray[ k ]
This.ScaleControl( cc.Hwnd , Scale , mode := cc.ScaleMode )
}
}
}
Re: Resize GUI with ALL its contents
Amazing, Thank you very much! 
